Using pipes to mutate texts in Vue 3
Now that I'm moving all my projects to Vue 3, I found something that I didn't like very much but it was easy to get used to, it's the issue of using the classic pipes to filter or mutate values that I used in Vue 2 and even in Angular I did it in a similar way.
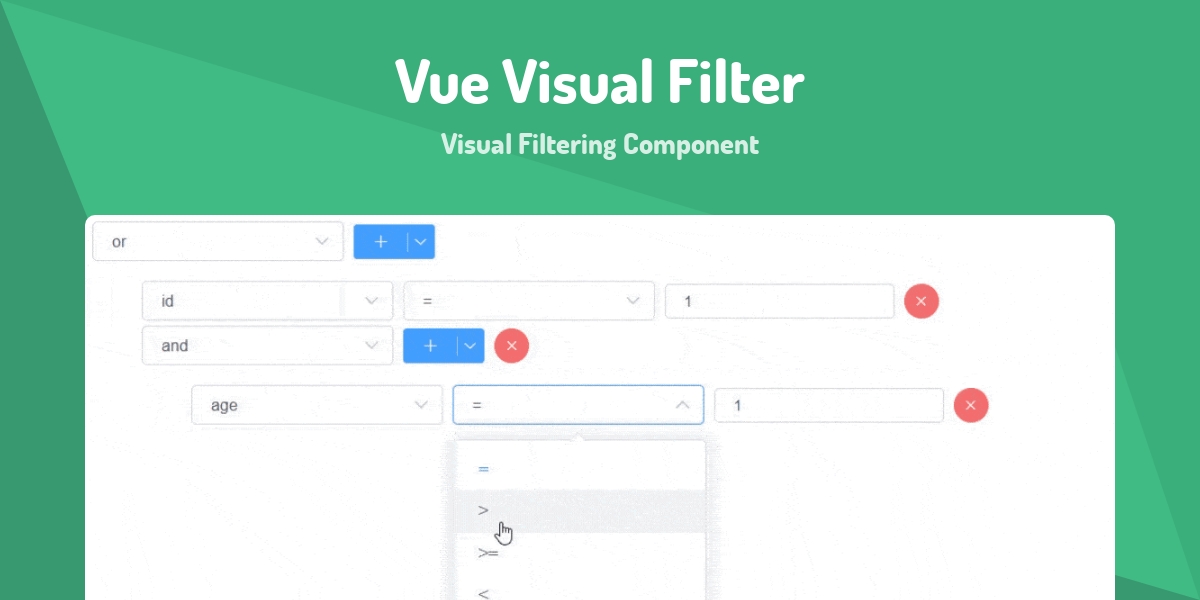
Now that I'm moving all my projects to Vue 3, I found something that I didn't like very much but it was easy to get used to, it's the issue of using the classic pipes to filter or mutate values that I used in Vue 2 and even in Angular I did it in a similar way.
Let me give you an example, when I had for example a date that came from an API and I wanted to mutate it so that it would be displayed in a nice way for the users in Vue 2, I used to use the "pipes" to create a general function that I could apply to all the parameters that shared that format in this way:
<template>
<h1>Mutate Date</h1>
<p>{{ myDate | dateforHumans }}</p>
</template>
<script>
export default {
data() {
return {
myDate: new Date()
}
},
filters: {
dateforHumans(myDate) {
return new Date(myDate).toLocaleString()
}
}
}
</script>
The “pipe” | humanDate did it all for me, but now in Vue 3 this option has been removed, and instead we have the filter option with which we must now apply the mutation as follows:
const humanDate = computed(() => {
return new Date(myDate).toLocaleString()
})
And in our template we directly invoke our value as a property
<p class="ml-2 text-xs text-gray-500 grow">
{{ humanDate }}
</p>
Although this may seem strange, it is easy to get used to using it, however, I find a problem with generalization and therefore find a particular solution that (in my opinion) may be more efficient, however I have not seen performance tests to know if it is or not a good idea, the thing would be to simply have a function that does the mutation and invoke it with the value from our code like this:
const humanDate = (myDate: string) => {
return new Date(myDate).toLocaleString()
}
And we invoke it from the template simply like this:
<p>
{{ humanDate(myDate) }}
</p>
I hope it helps you and if so, leave a comment. xD